윅스 (Wix) 코딩 강의 고급 (Advanced) - 장바구니에 추가하기 기능 만들기 (Add to Cart Gallery) - 윅스 쇼핑몰, 스토어 (Wix Stores)
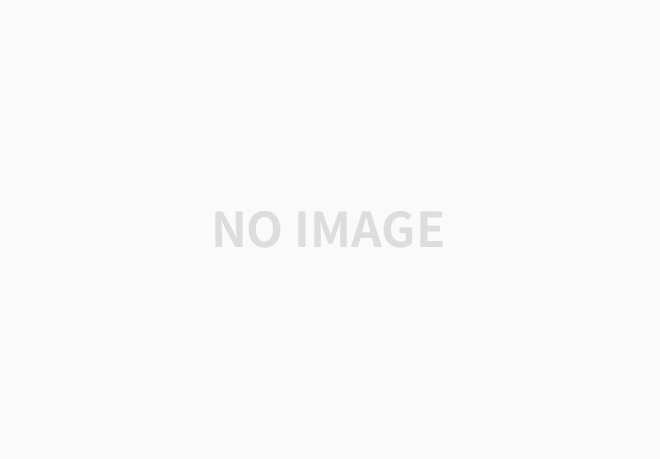
강의 내용 요약
다음의 예제는 Wix 윅스 무료 홈페이지 만들기의 자바스크립트 (Javascript) 코딩 기능을 활용할 수 있는 Corvid by Wix (윅스 코딩 - 콜비드) 를 활용하여 만듭니다.
웹사이트 쇼핑몰에 장바구니 추가하기 기능을 만듭니다.
Sunglasses | AddToCartGallery vw
www.wix.com
Log In | Wix
Login to Wix.com
users.wix.com
강의 내용 만드는법
만들고자 하는 윅스 사이트에 다음과 같은 구성 요소들이 필요합니다.
- 데이터베이스 컬렉션
- 이미지
- 텍스트
- 버튼
코드 (Code)
Sunglasses
//-------------Imports-------------//
import wixWindow from 'wix-window';
//-------------Global Variables-------------//
// Available colors.
const colorPicker = ['red', 'pink', 'yellow'];
// Map of product data.
let productsMap = {};
//-------------Repeater Setup-------------//
// Set up each item in the products repeater as it is loaded.
export function productsRepeater_itemReady($w, itemData, index) {
// Create a product object from the current item's data by calling the createProductObject() function.
let product = createProductObject(itemData);
// Add the newly created product to the global product map.
productsMap[product.productId] = product;
// Set the item's image to the main image from the product object.
$w('#image').src = product.mainImage.src;
// Set the item's price to the price from the product object.
$w('#price').text = product.price;
// Set the item's name to the name from the product object.
$w('#productName').text = product.name;
// If the site is being viewed on a mobile site:
if (wixWindow.formFactor === 'Mobile') {
colorPicker.forEach(color => {
$w(`#${color}`).show();
});
$w('#cartButton').show();
} else {
// Set the items's hover functionality by calling the initProductHover() function and passing it a selector scoped to the current item.
initProductHover($w);
// Set the item's direction hover functionality by calling the initDirectionsHover() function
// and passing it a selector scoped to the current item along with the current product object.
initDirectionsHover($w, product);
}
// Set the item's color picker functionality by calling the initColorPicker() function
// and passing it a selector scoped to the current item along with the current product object.
initColorPicker($w, product);
// Set the action that occurs when a user clicks the "Add to Cart" button for the current item.
$w('#cartButton').onClick(() => {
// Get the current product's object from the global products object.
let productObj = productsMap[product.productId];
// Add the current product to the shopping cart with the selected color.
$w("#shoppingCartIcon").addToCart(productObj.productId, 1, { choices: { Color: productObj.colorSelected } })
.then(() => console.log('added!'))
.catch(console.error);
});
}
//-------------Utility Functions for Repeater Setup-------------//
// Create an object that represents a project from the product data.
function createProductObject(productRecord) {
let product = {
colorSelected: 'red',
productId: productRecord._id,
productImagesByColor: {
red: {
front: productRecord.mediaItems[0],
right: productRecord.mediaItems[1],
left: productRecord.mediaItems[2]
},
pink: {
front: productRecord.mediaItems[3],
right: productRecord.mediaItems[4],
left: productRecord.mediaItems[5]
},
yellow: {
front: productRecord.mediaItems[6],
right: productRecord.mediaItems[7],
left: productRecord.mediaItems[8]
}
},
mainImage: productRecord.mediaItems[0],
price: productRecord.formattedPrice,
name: productRecord.name
}
return product;
}
// Initialize the product hover functionality.
function initProductHover($w) {
// Set the action that occurs when the mouse enters a product area.
$w('#productContainer').onMouseIn(() => {
// For each color defined in the global colorPicker variable:
colorPicker.forEach(colorChoice => {
// Show the color button.
$w(`#${colorChoice}`).show();
});
// Show the "Add to Cart" button.
$w('#cartButton').show();
});
$w('#productContainer').onMouseOut(() => {
// For each color defined in the global colorPicker variable:
colorPicker.forEach(colorChoice => {
// Hide the color button.
$w(`#${colorChoice}`).hide();
});
// Hide the "Add to Cart" button.
$w('#cartButton').hide();
});
}
// Initialize the color picker functionality for a product.
function initColorPicker($w, product) {
// For each color defined in the global colorPicker variable:
colorPicker.forEach(colorChoice => {
// Set the action that occurs when that color's corresponding button is clicked.
$w(`#${colorChoice}`).onClick(() => {
// Get the current product's object from the global productsMap variable.
let productObj = productsMap[product.productId];
// Set the product objects selected color to the color that was clicked.
productObj.colorSelected = colorChoice;
// Set the product image to the frontal image from the product object that corresponds to the color that was clicked.
$w('#image').src = productObj.productImagesByColor[colorChoice].front.src;
});
});
}
// Initialize the direction hover functionality for a product.
// Each product has a transparent box on the left third and right third of the product image.
function initDirectionsHover($w, product) {
// Local variables:
let productObj;
let colorSelected;
const directions = ['left', 'right'];
// For each direction in the directions list:
directions.forEach(direction => {
// Set the action that occurs when the user mouses into the box on that side of the image.
$w(`#${direction}Box`).onMouseIn(() => {
// Get the current product object.
productObj = productsMap[product.productId];
// Get the color that is currently displayed.
colorSelected = productObj.colorSelected;
// Set the product image to be the image for the current product
// with the selected color facing the direction that the mouse entered.
$w('#image').src = productObj.productImagesByColor[colorSelected][direction].src;
});
// Set the action that occurs when the user mouses out of the box on that side of the image.
$w(`#${direction}Box`).onMouseOut(() => {
// Get the current product object.
productObj = productsMap[product.productId];
// Get the color that is currently displayed.
colorSelected = productObj.colorSelected;
// Set the product image to be the image for the current product with the selected color facing forwards.
$w('#image').src = productObj.productImagesByColor[colorSelected].front.src;
});
});
}
Product Page
// For full API documentation, including code examples, visit http://wix.to/94BuAAs
$w.onReady(function () {
//TODO: write your page related code here...
});
Site Code
// For full API documentation, including code examples, visit http://wix.to/94BuAAs
$w.onReady(function () {
//TODO: write your page related code here...
});
API
Corvid by Wix (윅스 코딩 - 콜비드) 개발자 모드/도구 활성화하는 방법
윅스 (Wix) 코딩 - 개발자 도구를 활성화하기 (Wix Code: How to Enable Developer Tools)
윅스 (Wix) 코딩 - 개발자 도구를 활성화하기 (Wix Code: How to Enable Developer Tools)
윅스 (Wix) 코딩 - 개발자 도구를 활성화하기 (Wix Code: How to Enable Developer Tools) 설명) 개발자 도구를 활성화하기 위해서는 1. 에디터를 엽니다. 에디터 맨 위 상단 메뉴에서 코드를 클릭한 다음 개발�
limejuicer.tistory.com
연관된 토픽)
윅스 (Wix) 코딩 강의 고급 (Advanced) - 장바구니 기능 만들기 (Bulk Add to Cart) - 윅스 쇼핑몰, 스토어 (Wix Stores)
윅스 (Wix) 코딩 강의 고급 (Advanced) - 장바구니 기능 만들기 (Bulk Add to Cart) - 윅스 쇼핑몰, 스토어 (W
윅스 (Wix) 코딩 강의 고급 (Advanced) - 장바구니 기능 만들기 (Bulk Add to Cart) - 윅스 쇼핑몰, 스토어 (Wix Stores) 강의 내용 요약 다음의 예제는 Wix 윅스 무료 홈페이지 만들기의 자바스크립트 (Javascri..
limejuicer.tistory.com
윅스 홈페이지 만들기 101
윅스 (Wix) 홈페이지 만들기 101 - E-Book - Index
윅스 (Wix) 홈페이지 만들기 101 - E-Book - Index
윅스 (Wix) 홈페이지 만들기 101 - E-Book - Index 윅스 (Wix.com) 윅스 ADI & 템플릿 (Wix ADI & 템플릿) 윅스 웹에디터 (Wix Editor) 윅스 코딩 (Wix Code - Corvid) 윅스 해커톤 (Wix Hackathon)
limejuicer.tistory.com
출처 :
콜비드 - 윅스 코딩 (Corvid - Wix coding)
Add to Cart Gallery | Corvid by Wix Examples | Wix.com
In this example, we built a custom product gallery that lets a site visitor rotate a product’s image, select a product’s color, and add the selection to their cart.
www.wix.com
댓글